Python Programming
Overview
By the end of this course, students will be able to:
- Understand Python fundamentals, including variables, data types, and operators
- Write structured Python programs using conditionals, loops, and functions
- Work with data structures such as lists, tuples, dictionaries, and sets
- Handle file operations, error handling, and modular programming
- Use Python for basic data analysis and automation tasks
Build real-world projects demonstrating Python skills
Course Objectives
By the end of this course, students will be able to:
- Understand Python fundamentals, including variables, data types, and operators
- Write structured Python programs using conditionals, loops, and functions
- Work with data structures such as lists, tuples, dictionaries, and sets
- Handle file operations, error handling, and modular programming
- Use Python for basic data analysis and automation tasks
Build real-world projects demonstrating Python skills
Course Outline
Week 1 - Python Basics
- Installing Python and setting up an IDE (VS Code, PyCharm, or Jupyter Notebook)
- Writing and running your first Python script
- Python syntax, indentation, and comments
Variables, Data Types, and Operators
- Numbers, strings, booleans, lists, tuples, and dictionaries
- Arithmetic, assignment, comparison, and logical operators
- Type conversion and basic input/output operations
Week 2 - Control Flow - Conditions and Loops
- Conditional Statements (if, elif, else)
- Writing decision-making programs
- Nested conditions and logical operators
- Loops: For and While
- Iterating through lists and strings
- Using the range() function
Week 3 - Functions and Data Structures
- Functions in Python
- Defining and calling functions
- Parameters, arguments, and return values
- Scope (local vs. global variables)
- Working with Lists, Tuples, and Dictionaries
- List operations: append, remove, pop, slicing
- Tuple immutability and use cases
- Dictionaries: keys, values, adding/removing elements
Week 4 - File Handling and Error Handling
- Reading & Writing Files
- Opening, reading, writing, and closing files
- Working with CSV and text files
- Exception Handling
- Using try-except blocks
- Handling multiple exceptions
- Raising custom exceptions
Week 5 - Object-Oriented Programming (OOP) in Python
- Introduction to OOP Concepts
- Classes and objects
- Attributes and methods
- Constructor (__init__ method)
- Inheritance and Polymorphism
- Creating child classes
- Method overriding
Week 6 - Python for Real-World Applications
- Basic Data Analysis with Python
- Working with NumPy and Pandas
- Reading and analyzing datasets
- Final Project
Course Outcomes
By the end of this 6-week Python programming course, students will be able to:
Understand Python Fundamentals – Gain proficiency in Python syntax, variables, data types, and operators.
Write Structured Python Programs – Utilize conditional statements, loops, and functions to build efficient programs.
Work with Data Structures – Implement and manipulate lists, tuples, dictionaries, and sets.
Handle File Operations and Errors – Read/write files, work with CSV data, and manage exceptions for error-free execution.
Apply Object-Oriented Programming (OOP) – Understand classes, objects, inheritance, and polymorphism for structured programming.
Use Python for Data Analysis and Automation – Work with NumPy and Pandas for basic data manipulation and analysis.
Develop Real-World Projects – Apply learned concepts to create functional Python applications.
Expert Instructors
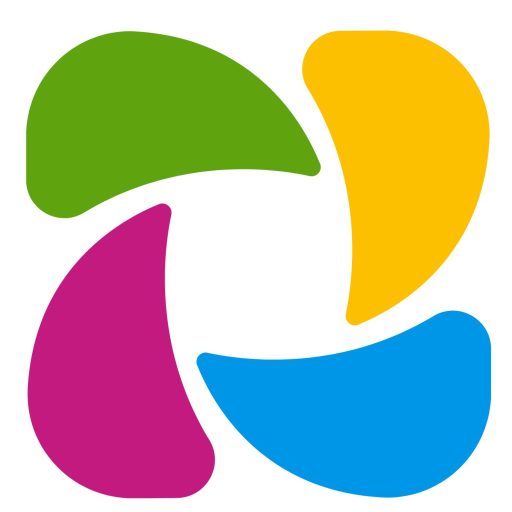
Owenosa S. Omogiate
Instructor
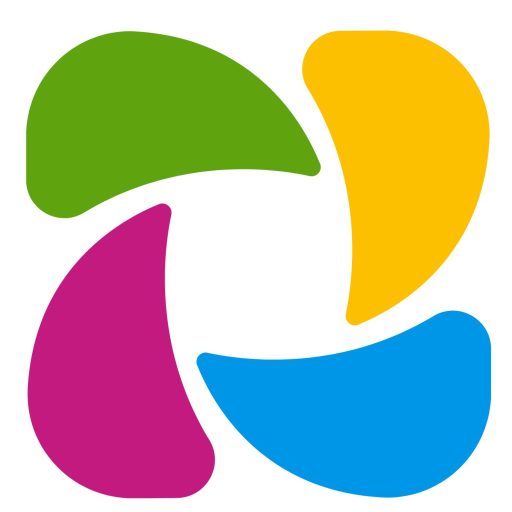
Daniel Ishaku
Instructor